Email functionality is a critical component for a vast array of web applications, from simple contact forms to complex notification systems. PHP, being a server-side scripting language, offers a built-in function for sending emails - the function. This guide dives deep into the nuances of using
, covering everything from basic usage to more advanced scenarios involving attachments and different content types. We'll also explore the setup requirements across different operating systems, the necessity of a remote mail server, and alternatives for reading and testing email functionalities in PHP.
Understanding the mail() Function in PHP
The function in PHP is a straightforward yet powerful tool for sending emails directly from a script. It constructs and sends a standard email, including headers and message body, using the local mail server or a specified SMTP server.
Basic Usage of mail()
Sending an email with the function involves specifying the recipient's email address, subject, message body, and additional headers as needed. Here's a simple example:
This snippet sends a basic email to with a simple message. The
header is used to specify the sender's email address.
A More Complex Example: Attachments and Multiple Content Types
Sending emails with attachments or mixed content types (e.g., combining text and HTML) requires constructing a multipart email. This involves specifying boundaries in the email body to separate different parts of the message, including the attachment data encoded in base64.
This example demonstrates sending an email with both text and an attachment, using MIME encoding and specifying content types and boundaries.
Setup Requirements for Using mail()
The function's behavior and capabilities depend significantly on the server configuration, particularly the settings in the
file and the underlying mail server setup (Sendmail on Unix/Linux or SMTP on Windows).
Configuring php.ini and Mail Server
-
On Linux/Unix (Sendmail): The
directive in
should point to the Sendmail executable. This is typically configured correctly by default on most Linux distributions.
-
On Windows (SMTP): Windows servers require specifying the SMTP server and port in
:
-
On macOS: Similar to Linux, macOS uses Sendmail or Postfix. Ensure the
is correctly set in
.
After adjusting , restart your web server for the changes to take effect.
Integrating the new sections into the previously outlined blog post, we enrich the guide with deeper insights into configuring email sending environments, specifically focusing on Sendmail and the Windows platform. These additions aim to provide a comprehensive understanding of the underlying systems that support PHP's function.
How email is sent by PHP
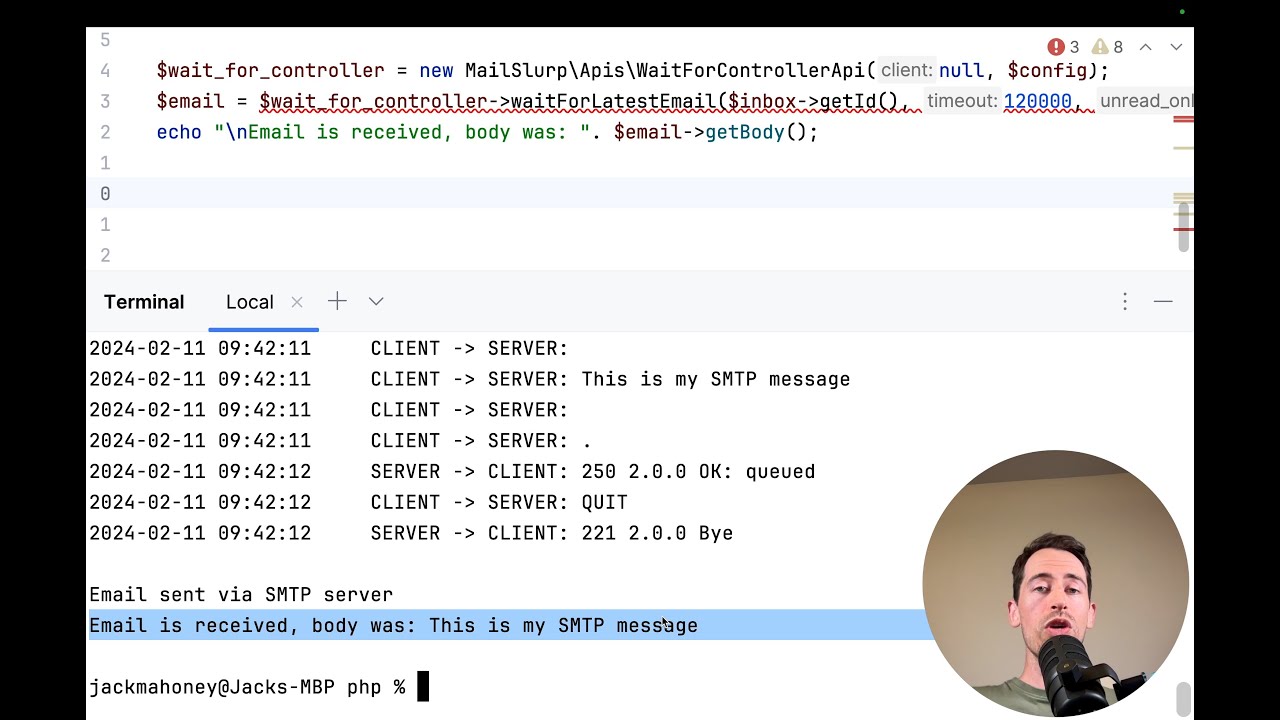
Depending on your platform, PHP will call either or, on Windows, use the built in SMTP system calls.
Sendmail is one of the oldest and most widely used mail transfer agents (MTA) on Unix-like operating systems. It's responsible for the routing and delivery of email. The PHP function can utilize Sendmail (or compatible MTAs like Postfix) for sending emails from scripts. On systems where Sendmail is not pre-installed or configured, manual setup is required to enable email sending capabilities.
Why Install Sendmail?
- Direct Mail Sending: Sendmail allows PHP applications to send emails directly from the server without relying on external SMTP services.
- Flexibility: It offers extensive configuration options for handling mail, including routing, authentication, and security measures.
- Compatibility: Sendmail's widespread support makes it a reliable choice for a variety of applications and server environments.
Setting Up and Using Sendmail
Installation on Linux
Most Linux distributions include Sendmail or an alternative like Postfix. To install Sendmail, you can use the package manager:
Basic Configuration
After installation, a basic configuration step is to ensure Sendmail is running and that PHP is configured to use it:
-
Start Sendmail:
-
Ensure PHP is Configured to Use Sendmail: This usually involves verifying the
directive in
points to the Sendmail executable. A typical setting might look like:
-
Test Sendmail: You can test Sendmail's functionality directly from the terminal:
This command sends a simple email to
, with verbose output to help diagnose any issues.
How Windows Sends Emails
Windows systems do not include Sendmail or a native equivalent. Instead, PHP on Windows uses SMTP to send emails. The configuration for this is done within the file, where you specify the SMTP server details that PHP should use:
For sending emails through SMTP on Windows, you need access to an SMTP server. This could be a service provided by your hosting company, an external email service like Gmail, or a local SMTP server running on your network. After configuring , restart your web server for the changes to take effect.
Additional SMTP Configuration for Windows
When using an external SMTP server, especially one that requires authentication, consider using a library like PHPMailer or SwiftMailer. These libraries offer a more straightforward approach to handling SMTP details, including authentication and encryption, which are not directly supported by PHP's function on Windows.
Why You Need a Remote Mail Server (SMTP) to Send Emails
While the function can send emails using a local mail server, using a remote SMTP server is often necessary for reliability, scalability, and security. A remote SMTP server handles email delivery, including queueing, retries, and bounce handling, more efficiently than a local setup, especially for applications with significant email traffic.
Reading Emails: IMAP and POP3 in PHP
PHP can interact with email servers not just to send but also to read emails using the IMAP and POP3 protocols. The function allows PHP scripts to connect to mail servers for reading messages, searching mailboxes, and managing emails programmatically. This capability is essential for applications that need to process incoming emails, such as support ticket systems.
Mocking and Testing mail() Usage in PHPUnit
Testing code that sends emails with can be challenging. To avoid sending actual emails during tests, you can mock the
function using PHPUnit's mocking capabilities. This involves creating a mock object that simulates the behavior of
without executing its real functionality, allowing you to verify that your code attempts to send an email under the right conditions.
Limitations of mail() and Alternatives
While is suitable for simple email sending tasks, its limitations become apparent with more complex requirements, such as SMTP authentication, encryption, and detailed error handling. Libraries like PHPMailer and SwiftMailer offer a more robust solution, providing comprehensive email sending features with support for SMTP, HTML emails, attachments, and more.
Conclusion
The PHP function serves as a basic tool for sending emails, but its simplicity comes with limitations. For production environments and applications with complex email sending needs, leveraging a dedicated library like PHPMailer or integrating with a professional SMTP service provides greater flexibility, reliability, and security. Regardless of the method chosen, understanding the underlying configuration and testing practices ensures that your application's email functionality meets its requirements effectively.